JavaScript is so flexible, and it allows you to call the same function in different manners. You can call the same function with ‘new’ keyword and without ‘new’ keyword. You can call the same function through an object and not through an object. How you call the function matters a lot and JavaScript would provide completely different results in each scenario.
Without understanding what happens under the hood, it’ll be difficult to debug too. In today’s blog post, we’re going to discuss the differences in calling a function with ‘new’ keyword and without ‘new’ keyword.
What would be the output of the below code snippet?
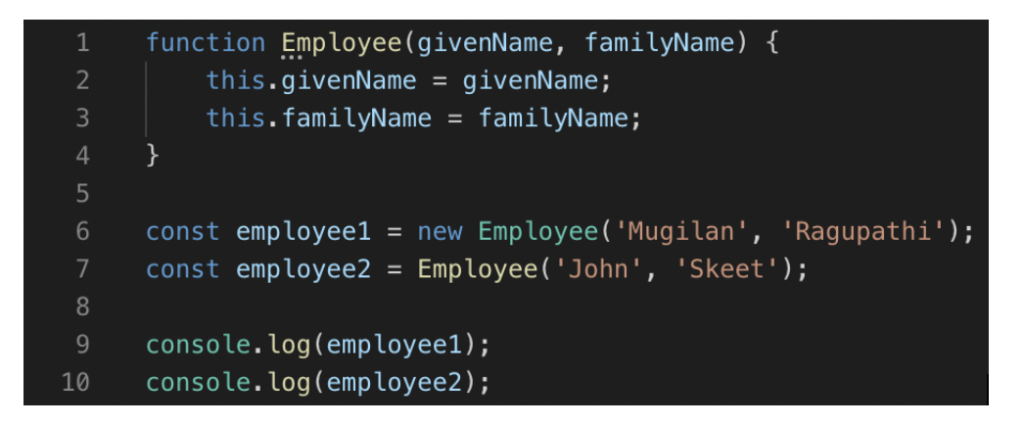
Take your time. THINK and answer.
The ‘console.log’ statement at line 9 would print – Employee {givenName: “Mugilan”, familyName: “Ragupathi”} and the console.log at line 10 would print undefined.
But why it behaves so? Let us analyse the above code snippet to truly understand what it does.
Lines from 1 to 4, we’re declaring a function by name ‘Employee’.
At line 6, we’re calling that function with new keyword.
Below things happen when you call a function with new keyword.
- JavaScript creates a new empty object. If ‘this’ keyword is used within that function, it refers to the newly created empty object.
- At line 2 of the above code snippet, javascript creates a property by name ‘givenName’ in the newly created object and assigns the value ‘Mugilan’(which is passed while calling the function). At line 3, same thing happens – javascript creates a property ‘familyName’ in the created object and assigns the value ‘Ragupathi’.
- If you’re not explicitly returning any object, JavaScript would return that newly created object.
Please note that the above 3 steps would happen ONLY when you call a function with ‘new keyword.
Sidenote: In fact, it also creates a prototypal link when you call a function with ‘new’ keyword. But we can discuss about this prototypal link in another blog post. This will not affect the understanding of the code snippet shown above.
Thus, at line 6, we will have an object with couple of properties ‘givenName’ and ‘familyName’. And the values for these properties would be ‘Mugilan’ and ‘Ragupathi’ respectively.
At line 7, we’re calling the same function without new keyword. If you’re calling a function without new keyword, the value of ‘this’ keyword would be the object through which the function is called. But as you might notice, at line 7, we’re not calling the function using any object. So, ‘this’ keyword would refer to the global context. At lines 2 and 3, JavaScript would try to set the value of the properties ‘givenName’ and ‘familyName’ in global context. In browser environment, the global context would be your window object.
As we’re not calling the function with ‘new’ keyword, javascript would not return any object implicitly. So, at line 2, the value of ‘employee2’ would be undefined.
So, ‘console.log’ statement at line 9 would print – Employee {givenName: “Mugilan”, familyName: “Ragupathi”} and the console.log at line 10 would print undefined.
Let’s add a couple of ‘console.log’ statements to the above code snippet. I’ve added statements at line 12 and 13. What would be the output of the those 2 ‘console.log’ statements?
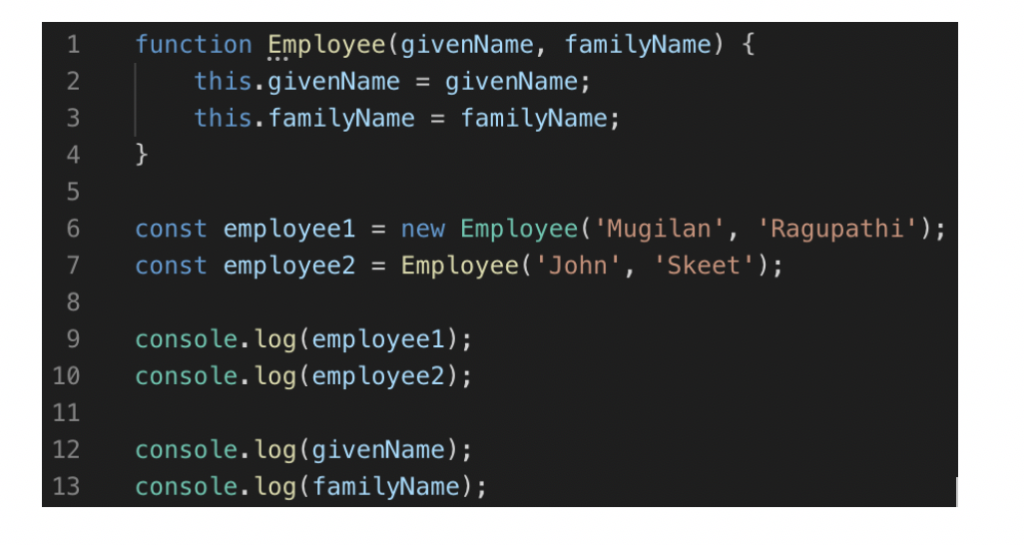
It would print ‘John’ and ‘Skeet’ respectively. When we were discussing about calling a function without ‘new’ keyword, we said that the properties would be created in the global context. At line 12 and 13, we’re accessing the properties ‘givenName’ and ‘familyName’ in global context, which is nothing but ‘window’ object in browser environment.
Let’s make one final change. Execute the code snippet in strict mode. At line 1, we’ve added a statement “use strict”, which executes our javascript code in strict mode. Now, what would be the output of the below code snippet.
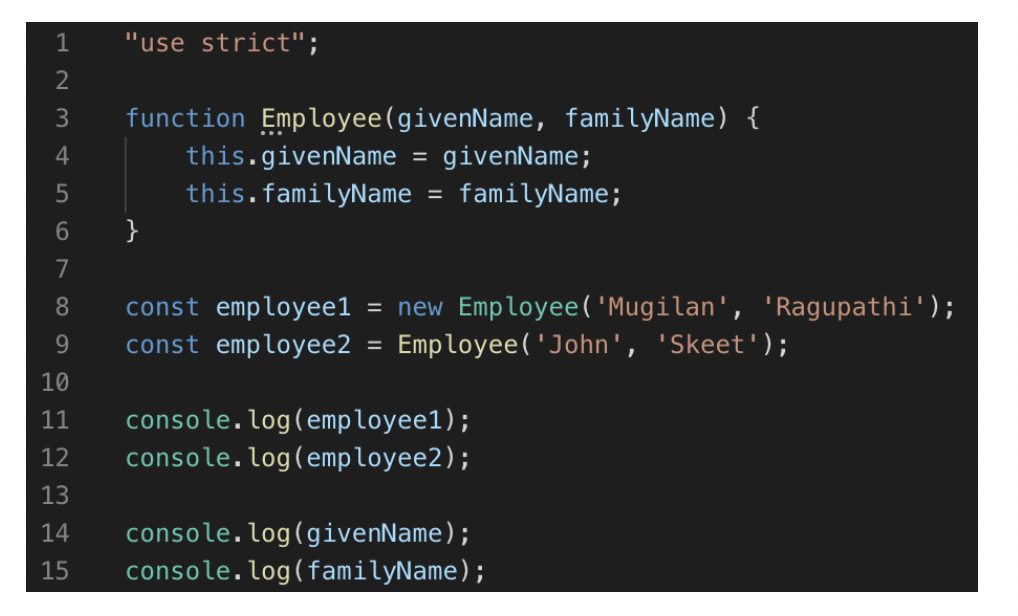
Answer: It will throw the error saying “Cannot set property
of undefined”. In strict mode, the global context is not window object and it
will be undefined. So, at line 2
(when you’re calling the function without new keyword at line 9), the value of
‘this’ keyword would still point to the global context and will have the value
of undefined. And when you try to set the property ‘givenName’ of undefined, it
throws error.
Hope you’ve understood the difference in calling the function with ‘new’ keyword and without ‘new’ keyword. Please let me know your thoughts in comments.